The Jones Calculus: Symbolic Algebra
Scott Prahl
April 2020
[1]:
#this must be run first
%matplotlib inline
import matplotlib
import numpy as np
import matplotlib.pyplot as plt
import sympy
import pypolar.sym_jones as sym_jones
sympy.init_printing(use_latex='mathjax')
Background
Jones Vector for Linearly Polarized Light
A Jones vector is a 2x1 matrix that can represent fully polarized light. Linearly polarized light is relatively simple
and
[2]:
sym_jones.field_horizontal()
[2]:
[3]:
sym_jones.field_vertical()
[3]:
So the vector for linearly polarized light at 45 degrees can be written in python as
[4]:
theta = sympy.Symbol('theta', real=True)
sym_jones.field_linear(theta)
[4]:
Jones Vectors for Circularly Polarized Light
For circularly polarized light, the direction of polarization rotates through an entire circle every wavelength, and retains a constant magnitude.
\[\begin{split}\mbox{right linearly polarized light}= {1\over\sqrt{2}}\left[\begin{array}{c} j\\ 1\\ \end{array}\right]\end{split}\]
[5]:
sym_jones.field_right_circular()
[5]:
So the vector for left circularly polarized light can be written in python as
[6]:
sym_jones.field_left_circular()
[6]:
Elliptically polarized light
In general, the expression for elliptically polarized light is
Irradiance
The scalar intensity of the beam is the square of the field \(E\cdot E^*\),
or
where \(A^*\) is the complex conjugate of \(A\).
Jones Matrix for Linear Polarizer
The Jones matrix representing a perfect horizontal polarizer is
The matrix representing a rotation through an angle \(\theta\) is
The matrix representing a linear polarizer oriented at an angle of \(\theta\) to the horizontal axis can by rotating the beam so the axis coincides with the horizontal axis and then unrotating by the same amount
[7]:
## By rotation
theta = sympy.Symbol('theta', real=True)
H = sym_jones.op_linear_polarizer(0)
R = sym_jones.op_rotation(theta)
linear = sym_jones.op_rotation(-theta) * H * sym_jones.op_rotation(theta)
linear
[7]:
[8]:
## Directly
sym_jones.op_linear_polarizer(theta)
[8]:
Retarders and Wave Plates
An optical retarder has a fast and a slow axis. Light polarized parallel to the fast axis moves through the wave plate faster than light polarized perpendicular to the fast axis. A phase lag (or retardance) \(\phi\) is created between the two polarization states. The Jones matrix for a retarder with its fast axis oriented at \(\theta\) is
[9]:
## are these the same??
phi = sympy.Symbol('phi', real=True)
sym_jones.op_retarder(theta,phi)
[9]:
[10]:
theta = sympy.Symbol('theta', real=True)
slm = sym_jones.op_retarder(sympy.pi/2,phi)
i0 = sym_jones.field_linear(sympy.pi/4)
anal = sym_jones.op_linear_polarizer(theta)
result = slm * i0
result/result[0]
[10]:
HaIf the thickness of the retarder is \(d\) and the fast and slow indices of refraction are \(n_o\) and \(n_e\), then the retardance for a wavelength \(\lambda\) is
A half-wave plate has \(\phi(\lambda)=\pi\) and a Jones matrix
[11]:
sympy.simplify(sym_jones.op_half_wave_plate(theta))
[11]:
A quarter-wave plate has \(\phi(\lambda)=\pi/2\) and
[12]:
## same??
sym_jones.op_quarter_wave_plate(theta)
[12]:
A retarder exhibits different retardances at a different wavelengths. Because \(n_o-n_e\) changes slowly with wavelength, the phase at \(\lambda_1\) of a wave plated designed for \(\lambda_0\) is
This is relevant because you will be using phase plates that are created for wavelengths other than 633nm.
Optical Isolator
Linearly polarized light passes through the polarizer and is converted to right hand circularly polarized light by the quarter wave plate. Upon reflection, this beam will change handedness, and upon passage back through the quarter wave plate it is linear again but orthogonal to the polarizer.
Malus’s Law
Show that the transmission of linearly-polarized light as it passes through a linear analyzer at an angle \(\theta\).
[13]:
incident = sym_jones.field_horizontal()
linear = sym_jones.op_linear_polarizer(theta)
result = linear * incident
intensity = sym_jones.intensity(result)
fraction = sympy.simplify(intensity)
fraction
[13]:
[14]:
theta = np.linspace(0, 180, 100)
plt.plot(theta, np.cos(np.radians(theta))**2)
plt.title('Malus\'s Law')
plt.xlabel( "Analyzer Angle (degrees)" )
plt.ylabel( "Transmission" )
plt.show()
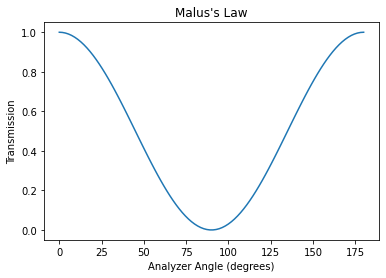
Crossed polarizers with quarter-wave plate between
Show that the total transmission of horizontally-polarized light that passes through a quarter-wave plate oriented at an angle \(\theta\) and then through a vertical analyzer is \(2\cos^2\theta\sin^2\theta\) and plot the transmitted light as the polarizer is rotated through 180 degrees.
[15]:
theta = sympy.Symbol('theta', real=True)
incident = sym_jones.field_horizontal()
plate = sym_jones.op_quarter_wave_plate(theta)
analyzer = sym_jones.op_linear_polarizer(sympy.pi/2)
result = analyzer * plate * incident
fraction = sym_jones.intensity(result)
fraction
[15]:
[16]:
theta = np.linspace(0, 180, 180)
th = 2*np.radians(theta)
plt.plot(theta, 0.5*np.sin(th)**2)
plt.title('Horizontal -> Quarter Wave Plate -> Vertical Analyzer')
plt.xlabel( "Quarter Waveplate angle (degrees)" )
plt.ylabel( "Transmission" )
plt.show()
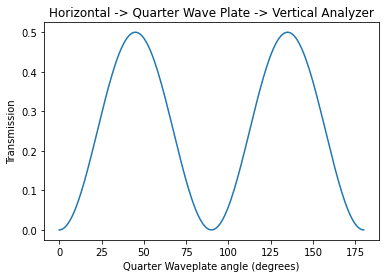
Crossed polarizers with half-wave plate between
Show that the total transmission of horizontally-polarized light that passes through a half-wave plate and then through a vertical linear analyzer is \(4\sin^2\theta\cos^2\theta = \sin^2 2\theta\) and plot as the analyzer is rotated through 180 degrees.
[17]:
theta = sympy.Symbol('theta', real=True)
incident = sym_jones.field_horizontal()
plate = sym_jones.op_half_wave_plate(theta)
analyzer = sym_jones.op_linear_polarizer(sympy.pi/2)
result = analyzer * plate * incident
fraction = sym_jones.intensity(result)
fraction
[17]:
[18]:
theta = np.linspace(0, 180, 500)
plt.plot(theta, np.sin(np.radians(2*theta))**2 )
plt.title('Horizontal -> Half Wave Plate -> Vertical Analyzer')
plt.xlabel( "Half waveplate Angle (degrees)" )
plt.ylabel( "Transmission" )
plt.show()
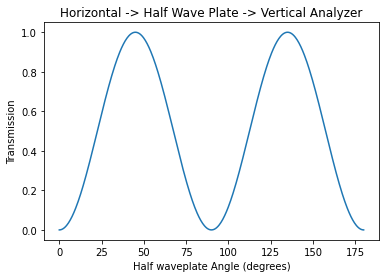
Waveplates not at design wavelength
The phase shift in a halfwave plate depends on the wavelength. If the halfwave plates are designed for 532nm light, but we use them for 633nm light then how will the measurements be affected? (Assume the speeds along the fast and slow axis are independent of wavelength).
Plot the transmitted light for both 633 and 532nm light.
[19]:
f = sympy.Symbol('f', real=True)
theta = sympy.Symbol('theta', real=True)
lambda_0 = sympy.Symbol('lambda_0', real=True)
lambda_1 = sympy.Symbol('lambda_1', real=True)
f = lambda_0/lambda_1
incident = sym_jones.field_horizontal()
plate_1 = sym_jones.op_retarder(theta,f*sympy.pi)
analyzer = sym_jones.op_linear_polarizer(sympy.pi/2)
result_1 = analyzer * plate_1 * incident
fraction_1 = sym_jones.intensity(result_1)
fraction_1
[19]:
[20]:
lambda_0 = 532e-9
lambda_1 = 633e-9
theta = np.linspace(0, 180, 500)
plt.plot(theta, np.sin(np.radians(2*theta))**2 ,label='532nm')
plt.plot(theta, np.sin(lambda_0*np.pi/2/lambda_1)**2*np.sin(np.radians(2*theta))**2, label='633nm' )
plt.title('Horizontal -> Half Wave Plate -> Vertical Analyzer')
plt.xlabel( "Half waveplate Angle (degrees)" )
plt.ylabel( "Transmission" )
plt.legend()
plt.show()
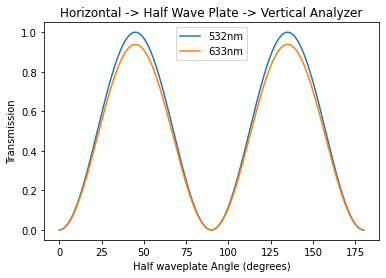